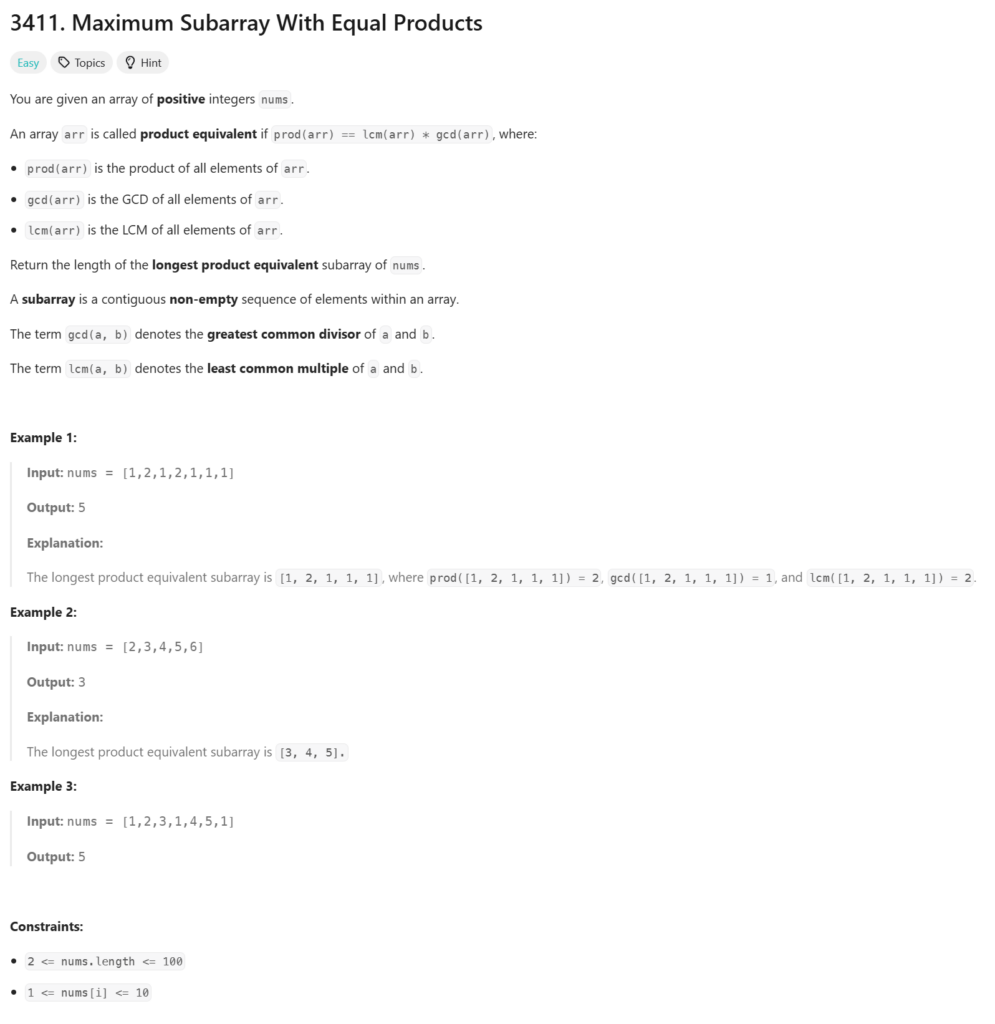
You are given an array of positive integers nums.
An array arr
is called product equivalent if prod(arr) == lcm(arr) * gcd(arr)
, where:
prod(arr)
is the product of all elements ofarr
.gcd(arr)
is the GCD of all elements ofarr
.lcm(arr)
is the LCM of all elements ofarr
.
Return the length of the longest product equivalent subarray of nums
.
Constraints:
- 2 <= nums.length <= 100
- 1 <= nums[i] <= 10
GCD (Greatest Common Divisor) means the largest number that can divide both numbers without leaving a remainder.
For example:
GCD of 12 and 18 is 6 because 6 is the largest number that can divide both 12 and 18 exactly (12 ÷ 6 = 2, 18 ÷ 6 = 3).
In python, we can use these two methods for getting gcd of 2 numbers:
def gcd(a, b):
while b:
a, b = b, a % b
return a
def gcd(a, b):
return math.gcd(a, b)
LCM (Least Common Multiple) means the smallest positive number that is a multiple of both numbers.
For example:
LCM of 12 and 18 is 36, because:
36 is divisible by both 12 and 18.
It is the smallest number that satisfies this condition.
Relationship Between GCD and LCM:
lcm(a, b) = abs(a * b) / gcd(a, b)
class Solution:
def maxLength(self, nums: List[int]) -> int:
n = len(nums)
ans = 1
for i in range(n):
p = nums[i]
g = nums[i]
l = nums[i]
for j in range(i + 1, n):
p *= nums[j]
g = math.gcd(g, nums[j])
l = self.lcm(l, nums[j])
if l * g == p:
ans = max(ans, j - i + 1)
return ans
def lcm(self, a, b):
return abs(a * b) // math.gcd(a, b)
Extremally difficult to use wp to post a blog.